Last Update:
Let's Talk About String Operations in C++17
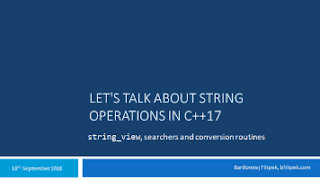
Table of Contents
In September our local C++ User Group started a “new year” of meetings after a little break in August. I had a pleasure to give a talk about string operations in C++17.
Here are the slides and additional comments.
The Talk
For my book I wrote a lot of content about string_view
,
std::searcher
and std::to_chars
, std::from_chars
and I wanted to
make a short summary of those features.
In the talk I included some of my benchmarks and notes that were covered in my blog, for example:
- Preprocessing Phase for C++17’s Searchers
- Speeding up Pattern Searches with Boyer-Moore Algorithm from C++17
- Speeding Up string_view String Split Implementation
- Performance of std::string_view vs std::string from C++17
- plus the new content about conversion routines.
Most of the time we spent discussing string_view
as this feature might
have a bigger impact on your code. std::searcher
and low-level
conversion routines are quite specialized so they won’t be used that
often as views.
For example, during the discussion, we shared the experience using
string views. One case is that when you refactor some existing code
you’ll often find a situation where you can use views through chains of
function calls, but then, at some point, you’re stuck as you have to
perform conversion to string
anyway.
Another thing, that was brought by Andrzej Krzemieński (from Andrzej’s
C++ Blog). While string_view
is
supposed not to allocate any extra memory, you should be still prepared
for memory allocations for exceptions.
Have a look at this code:
#include <iostream>
#include <stdexcept>
#include <string_view>
void* operator new(std::size_t n)
{
std::cout << "new() " << n << " bytes\n";
return malloc(n);
}
int main()
{
std::string_view str_view("abcdef");
try {
for (std::size_t i = 0; true; ++i)
std::cout << i << ": " << str_view.at(i) << '\n';
}
catch (const std::out_of_range& e) {
std::cout << "Whooops. Index is out of range.\n";
std::cout << e.what() << '\n';
}
}
Play @Coliru
The code uses str_view.at(i)
that can throw when trying to access an
index out of range. When an exception is created, you’ll see some memory
allocation - for the message string.
It’s probably not super often to use at
, but it’s an interesting
observation.
The Slides
Let’s talks about string operations in C++17 from Bartlomiej Filipek
Summary
The talk was my third presentation for the Cracow User Group. It’s an amazing experience, and I hope to be able to deliver more good stuff in the future :)
What is your experience with string views, searchers and low-level conversion routines? Have you played with the new features?
I've prepared a valuable bonus for you!
Learn all major features of recent C++ Standards on my Reference Cards!
Check it out here: